External licensing systems
If you have pre-existing software that relies on other licensing systems than Moonbase, you can integrate the two. We support two main paths to achieve this integration:
- Using pre-uploaded key codes
- Using a HTTP API generator
To make it easier to transition between systems, our HTTP API generator supports several protocols:
- A modern JSON-based API integration with HMAC security signatures
- Compatibility modes for popular platforms like Fastspring, MyCommerce, DigitalRiver, ShareIt and others
If you are building APIs for your own licensing backend, using our JSON-based integration is highly recommended, but if you are transitioning from other payment providers, our compatibility mode might let you move over without any changes necessary in your backend systems.
Do you have a specific scenario you need to support or other questions?
Reach out to us through the support channel, or at developers@moonbase.sh.
Pre-uploaded key codes
In the case your licensing system can pre-generate arbitrary key codes for activating your software, you may opt to use our key code list feature. To get started with this, simply choose the "Key Codes" generator on your product in Moonbase:
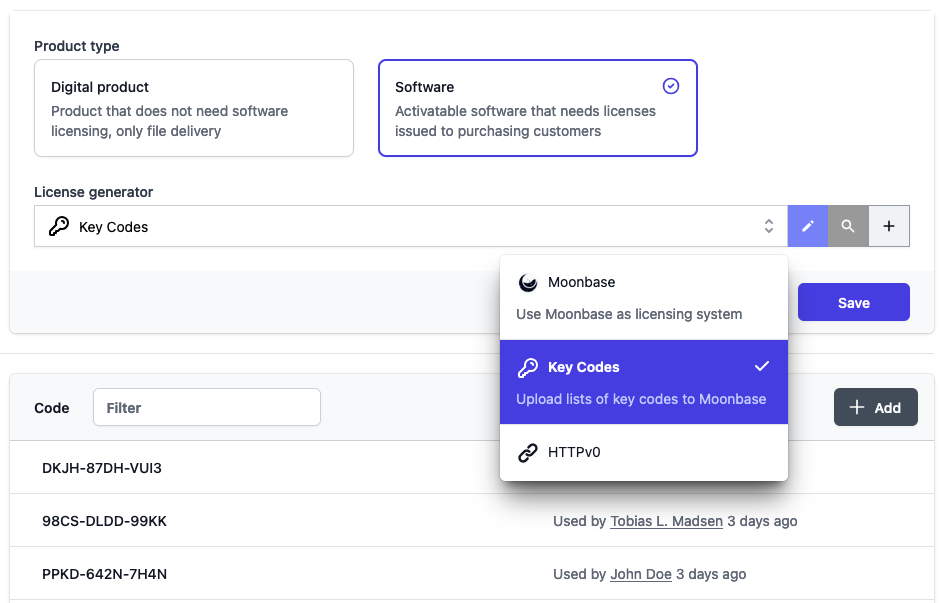
At this point, you may start uploading as many key codes as you wish to be used for future purchases. The product overview will indicate whether or not a product has enough key codes remaining, and it's your responsibility to ensure codes don't run out.
HTTP generator
Using our HTTP generator means that Moonbase will call your API on customer purchases to fulfill the order with licenses. It's important that your API is always available, so that customers will get access to products when they make their payment. The API call has a timeout of 5 seconds, and will retry up to 3 times if a failure code is returned.
Your endpoint should be able to handle the request payload, and return a JSON object with licenses per product:
Request payload
- Name
type
- Type
- enum(Order|Voucher|License)
- Description
The type of fulfillment being requested,
Order
being purchases,Voucher
being voucher redemptions andLicense
being manual license provisioning from the Moonbase app.
- Name
reference
- Type
- string
- Description
A reference to the Order/Voucher/License being fulfilled, this will be unique across all fulfillment requests your backend may receive.
- Name
customer
- Type
- object
- Description
Details about the customer who initiated the fulfillment, it may have the following fields:
- Name
id
- Type
- string
- Description
Globally unique customer ID for this customer.
- Name
name
- Type
- string
- Description
Full name of the customer.
- Name
businessName
- Type
- string
- Nullability
- nullable
- Description
If the customer is a business, this will have the name of the business.
- Name
taxId
- Type
- string
- Nullability
- nullable
- Description
If the customer is a business, this will have the tax ID of the business if given.
- Name
email
- Type
- string
- Description
Email address of the customer, this is always present and used as the login for the customer. Do note that Moonbase supports customers changing the email address of their account.
- Name
address
- Type
- object
- Nullability
- nullable
- Description
The billing address of the customer if given:
- Name
countryCode
- Type
- string
- Description
ISO 3166-1 alpha-2 two-letter country code.
- Name
streetAddress1
- Type
- string
- Description
First line of the regular street address.
- Name
streetAddress2
- Type
- string
- Optionality
- optional
- Description
Second line of the regular street address.
- Name
postCode
- Type
- string
- Description
Postal code of the address.
- Name
locality
- Type
- string
- Description
Locality of the address, only required if no region is given.
Also known asCity
.
- Name
region
- Type
- string
- Description
Region of the address, only required if no locality is given.
Also known asState
.
- Name
requests
- Type
- array<object>
- Description
A list of license requests with objects of the following shape:
- Name
productId
- Type
- string
- Description
The ID of the product that needs licenses.
- Name
quantity
- Type
- number
- Description
The number of licenses that is requested.
Request
POST https://license-backend.your-domain.example/fulfill
Content-Type: application/json
X-Signature: HNA0AVKUMOF5CXC8GKELYVYTYK37Q4ONGLJEL2TJTGA=
{
"type" : "Order",
"reference" : "b28f721c-c29a-4fc4-9153-04f128283c51",
"customer" : {
"id" : "2d473e34-fc59-4e6e-9f38-4ad379baa8e9",
"name" : "John Doe",
"businessName" : null,
"taxId" : null,
"email" : "john.doe@example.com",
"address" : null
},
"requests" : [
{
"productId" : "example-product",
"quantity" : 2
}
]
}
Expected response
When responding to license requests, your backend should respond with a dictionary where the key is the product ID being fulfilled, and the value an array of licenses to issue to the customer.
Each license string may contain line feed characters, but should not contain any sort of rich text content or HTML.
It's imperative that the number of values in the list matches the requested quantity of licenses for the given product.
Response
{
"example-product" : [
"LICENSE-KEY-1",
"LICENSE-KEY-2"
]
}
Security
To ensure your fulfillment endpoint is secure and safe from request forgery, we attach a signature to every fulfillment request being sent. This signature is using a HMAC-SHA256 authentication code using the secret set up while configuring the generator in the Moonbase app. Using the raw request body and the secret, you can calculate a signature, and make sure that the signature you calculate is the same as ours.
Example signature verification code
using var algorithm = new HMACSHA256(Encoding.UTF8.GetBytes(expectedSecret));
var json = await request.Content.ReadAsStringAsync();
var hash = algorithm.ComputeHash(Encoding.UTF8.GetBytes(json));
var signature = Convert.ToBase64String(hash).ToUpperInvariant();
var sentSignature = request.Headers.GetValues("X-Signature").Single();
Assert.Equal(sentSignature, signature);
If you're using out compatibility modes for other platforms, security patterns may differ. Reach out to us for more information about maintaining secure endpoints for license fulfillment.