Embedded Storefront
The fastest path to a native look and feel for your Moonbase-powered e-commerce is using our embedded storefront package. It comes with pre-built UI for customer authentication, cart management, checkout flow, license activations and much more. Although the UI is included, we've exposed a number of style variables so that you can make it yours.
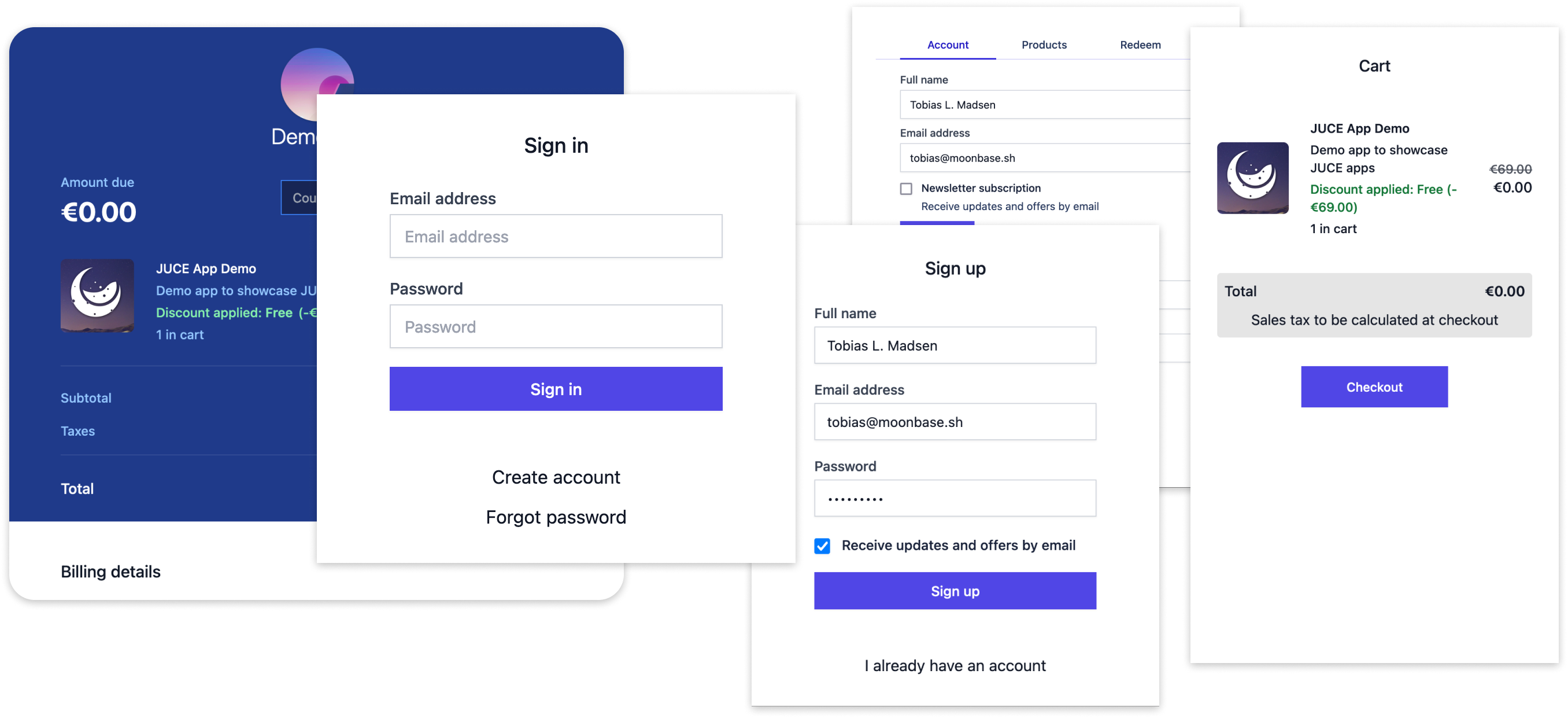
This package can be included in your own websites, no matter what platform you use. On this page you will find instructions for popular platforms, but if yours is missing, reach out to us through the support channel, or at developers@moonbase.sh.
To make sure the module works on your website, take care to add your domain to the whitelisted domains of your Moonbase account in your account settings. If you experience CORS errors, the domain is not whitelisted correctly. Once live, you should also switch the storefront mode of your Moonbase account to direct customers to your website instead of the Moonbase hosted customer portal.
Getting started
To add e-commerce capabilities to your website, install using the appropriate method:
Static websites
For static websites, a simple <script>
element is enough to add the basic features:
<script type="module" src="https://assets.moonbase.sh/storefront/moonbase.js"></script>
<script type="text/javascript">
document.addEventListener('DOMContentLoaded', () => {
// TODO: Replace this with the URL of your Moonbase account
Moonbase.setup('https://demo.moonbase.sh')
})
</script>
This snippet can be added at the end of your document, and should be included on every page you want storefront features available on. Read on for more details on how to configure buttons and render dynamic content.
Javascript apps
To make it easier to use this when building sites using React.js, Vue.js, Svelte, or similar frameworks, you can also add this package to your dependencies and install it:
npm install @moonbase.sh/storefront --save
At this point you can import and call the Moonbase setup method whenever your UI is ready:
import Moonbase from '@moonbase.sh/storefront'
// TODO: Replace this with the URL of your Moonbase account
Moonbase.setup('https://demo.moonbase.sh')
The package comes with full Typescript support and supports all the features listed below.
Be sure to keep this package updated, as we often publish improvements and bug fixes to optimize your storefront.
Wordpress
In case you're running a Wordpress site, you can install the Moonbase plugin on your instance. This plugin will add the necessary scripts to your pages, and also give you the option to insert "Add to cart" buttons as part of your content, either using short codes, or as blocks in your content. The features of this plugin is quite minimal; if you need more extensive customization you should edit your theme directly.
Website builders
For other website builders, we've written some articles on how to get started:
Squarespace is a great way to make websites fast and easy, and can be combined with Moonbase to enabled advanced e-commerce capabilities. This article shows you how to get started.
Customize the experience
Everything from here on is optional, and only required if you need customizations.
Look & Feel
The Moonbase embedded storefront is themeable to some degree; you may tweak the brand color, select fonts, style buttons and cards, and more.
This is done using the theme
property of the options given when first setting up the module:
{
theme: {
dark: true | false,
colors: {
primary: '#1A77F2',
background: 'white' | 'gray',
},
fonts: {
heading: 'Poppins' | 'PT Serif' | 'Montserrat' | 'Aleo',
body: 'Inter' | 'Roboto' | 'EB Garamond' | 'Merriweather',
},
corners: 'sharp' | 'soft' | 'round',
buttons: 'outlined' | 'light',
cards: 'outlined' | 'shadow' | 'white',
}
}
Any further customizations done by your website is not officially supported.
If you are noticing styles from your own website bleed in to the Moonbase storefront, then try to avoid any !important
style rules on your own website.
Configure options
When adding the package to your site, you can configure other parts of the experience by overriding any the settings listed below, this is their default values:
{
toolbar: {
// Whether or not to show the toolbar
enabled: true,
// The location of the toolbar, can be one of:
// `top-right`, `top-left`, `bottom-right`, `bottom-left`
location: 'top-right',
show: {
// Whether or not to show the cart button of the toolbar
cart: true,
// Whether or not to show the account button of the toolbar
account: true,
// Whether or not to show the Moonbase logo in the toolbar
moonbase: true,
},
},
auth: {
signIn: {
// Enables or disables customer log-ins
enabled: true,
// Hint can be a string with text to show during sign in
hint: undefined,
},
signUp: {
// Enables or disables customer sign-ups
enabled: true,
},
},
checkout: {
// Changes the checkout flow to be a redirect based flow
redirect: false,
},
activation: {
// Determines what device token files should be uploadable
deviceTokenFileExtension: '.dt',
// Sets the name of the downloaded liense token file
licenseTokenFileName: 'license-file.mb',
},
theme: {
// See the above section for details on look and feel
},
}
To override them, simply pass in options with your configuration when setting up the storefront:
<script type="module" src="https://assets.moonbase.sh/storefront/moonbase.js"></script>
<script type="text/javascript">
document.addEventListener('DOMContentLoaded', () => {
// TODO: Replace this with the URL of your Moonbase account
Moonbase.setup('https://demo.moonbase.sh', {
toolbar: {
// We have custom buttons to trigger account and cart,
// so let's hide the default toolbar.
enabled: false,
}
})
})
</script>
Call methods
The Moonbase embedded storefront supports a whole range features, from authentication, to e-commerce and licensing. By default, it will pick up on URL parameters that contains intents, but you can also trigger these yourself. Below is the full list of intents you can initiate:
type MoonbaseInstance = {
// Identity
sign_in(parameters?: { email?: string; }): void
sign_up(parameters?: { email?: string; }): void
forgot_password(parameters?: { email?: string; }): void
reset_password(parameters: { email: string; code: string; }): void
confirm_account(parameters: { email: string; code: string; }): void
confirm_email(parameters: { email: string; code: string; }): void
confirm_email_change(parameters: { email: string; code: string; }): void
// Customer
view_account(): void
view_products(): void
redeem_voucher(parameters?: { code?: string; }): void
// Products
view_product(parameters: { product_id: string; version?: string; }): void
activate_product(parameters?: { token?: string; }): void
// Orders
view_cart(): void
add_to_cart(parameters?: {
product_id?: string;
bundle_id?: string;
variation_id?: string;
quantity?: number;
}): void
purchase(parameters?: {
product_id?: string;
bundle_id?: string;
variation_id?: string;
quantity?: number;
} | {
product_id?: string;
bundle_id?: string;
variation_id?: string;
quantity?: number;
}[]): void
checkout(parameters?: { complete?: boolean; }): void
close_checkout(): void
}
To actually trigger the UI elements, you can add onclick handlers to buttons like so:
<button onclick="Moonbase.add_to_cart({ product_id: 'example-product' })">
Add to cart
</button>
<button onclick="Moonbase.purchase({ bundle_id: 'example-bundle', quantity: 2 })">
Buy now
</button>
Or, if using the Javascript package, you can also call them from your code:
import Moonbase from '@moonbase.sh/storefront'
const onAddToCartButtonClick = () => {
Moonbase.add_to_cart({ product_id: 'example-product' })
};
Render dynamic content
The embedded storefront will fetch and cache data related to products & bundles, the authenticated customer, and the current cart. To make your web site dynamic, we support rendering and conditionally hiding/showing elements part of your site.
Rendering content is as simple as adding the data-moonbase-render
attribute to the elements you want dynamic content to appear in:
<button data-moonbase-render="user.name" onclick="Moonbase.view_account()">
Account
</button>
Any initial content will be replaced with data loaded by the embedded storefront, if present. In the above example, the button will show "Account" until a customer signs in, after which it will show the user's name.
The available properties to render are:
# User properties
user.name
user.email
# Cart properties
cart.item_count
# Product properties
product.<product_id>.name
product.<product_id>.price
product.<product_id>.original_price
product.<product_id>.discount_name
product.<product_id>.discount_description
product.<product_id>.discount_total
# Bundle properties
bundle.<bundle_id>.name
bundle.<bundle_id>.price
bundle.<bundle_id>.original_price
bundle.<bundle_id>.discount_name
bundle.<bundle_id>.discount_description
bundle.<bundle_id>.discount_total
# You can also access data for specific pricing variations:
product.<product_id>.variation[<variation_id>].price
product.<product_id>.variation[<variation_id>].original_price
product.<product_id>.variation[<variation_id>].discount_name
product.<product_id>.variation[<variation_id>].discount_description
product.<product_id>.variation[<variation_id>].discount_total
Using these, you can enrich your static websites with dynamic data based on the current pricing configured in Moonbase, as well as the context of the customer currently logged in, including segmented discounts they may have access to.
In case you have elements you want to only conditionally show based on properties, we support a data-moonbase-if
attribute:
<button
hidden
data-moonbase-if="user"
data-moonbase-render="user.name"
onclick="Moonbase.view_account()"
></button>
Any elements with data-moonbase-if
attributes will have their hidden
attribute set based on storefront context.
In the above example, we add the hidden
attribute to ensure it's hidden by default, and only when a user is authenticated will is show.
The available properties to conditionally render based on are:
# User properties
user
# Cart properties
cart.has_items
# Product properties
product.<product_id>
product.<product_id>.has_discount
product.<product_id>.has_discount_description
# Bundle properties
bundle.<bundle_id>
bundle.<bundle_id>.has_discount
bundle.<bundle_id>.has_discount_description
Listen for events
In case you want to react to the storefront library being used, either for analytics or other purposes, you can register event listeners. These listeners work much like native HTML event listeners, and you can register them on the Moonbase instance directly:
import Moonbase from '@moonbase.sh/storefront'
Moonbase.on('checkout-completed', event => console.log('Purchase completed:', event.order))
These are all the available events you can currently listen for:
// Identity related events
'signed-in': {
user: User
}
'signed-up': {
user: User
}
'signed-out': {
user: User
}
// Voucher related events
'redeemed-voucher': {
voucher: Voucher
user: User
}
// Product related events
'downloaded-product': {
product: OwnedProduct
download: Download
user?: User | null
}
'activated-product': {
product: StorefrontProduct
fulfillmentType: ActivationRequestFulfillmentType
user?: User | null
}
// Cart related events
'added-to-cart': {
item: CartItem
currency: string
user?: User | null
}
'checkout-initiated': {
order: Order
user?: User | null
}
'checkout-closed': {
order: Order
user?: User | null
}
'checkout-completed': {
order: Order
user?: User | null
}
To see a practical example of how to use these for analytics, check out these articles:
See how you can hook up the embedded storefront to send e-commerce events to Google Analytics
If you are doing Facebook, Instagram or WhatsApp marketing, you should be enriching your Meta Pixel events with actions done by customers in the embedded storefront, learn more in this article.